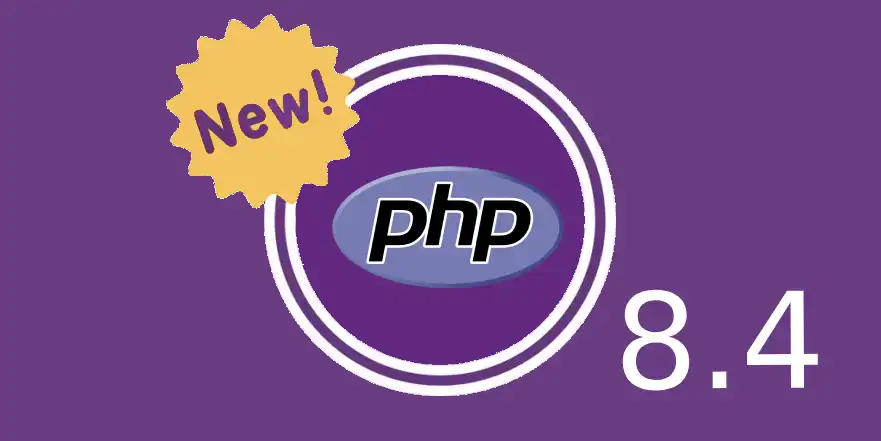
Introduction
PHP 8.4 is a significant release that brings a host of exciting new features and improvements designed to enhance developer productivity. With additions like property hooks, asymmetric visibility, and advanced array functions, PHP 8.4 empowers developers to write cleaner, more efficient code. Beyond these core updates, PHP 8.4 also introduces performance optimizations, new tools for working with dates, strings, and HTML5, along with simplifications like creating objects without parentheses. Whether you're optimizing legacy code or starting new projects, PHP 8.4 offers the flexibility and power for modern development. Let’s explore the key features and how they can benefit your development process.
1. Property Hooks
Property hooks enable you to define custom logic for reading and writing property values directly in the property declaration, reducing boilerplate code. For example, you can implement rules for setting and getting properties like this:
class Person {
public int $age with get, set;
public function getAge(): int {
return $this->$age * 2; // Double the age
}
public function setAge(int $age): void {
if ($age < 0) {
throw new InvalidArgumentException('Age cannot be negative');
}
$this->$age = $age;
}
}
$person = new Person();
$person->$age = -5; // This will throw an exception
echo $person->$age; // Outputs double the actual age
2. Asymmetric Visibility
This feature allows you to set different visibility levels for class properties and methods. For example, you can have a public property but a private method to modify it:
class MyClass {
public int $publicProperty;
private int $privateProperty;
public function setPrivateProperty(int $value): void {
$this->$privateProperty = $value;
}
}
In this example, $publicProperty can be accessed from anywhere, but $privateProperty can only be accessed within the class itself.
3. New Array Functions
PHP 8.4 introduces new array functions that make working with arrays easier. Some of these include:
- array_is_list: Checks if an array is a list (i.e., has integer keys starting from 0).
- array_merge_recursive_distinct: Merges arrays recursively, merging values with the same key and preserving distinct ones.
- array_unique_recursive: Removes duplicate values from an array, even in nested arrays.
Here’s an example using array_merge_recursive_distinct:
$array1 = ['a' => 1, 'b' => ['c' => 2]];
$array2 = ['a' => 2, 'b' => ['d' => 3]];
$mergedArray = array_merge_recursive_distinct($array1, $array2);
Other Key Features in PHP 8.4
- Performance Improvements: The Just-In-Time (JIT) compiler has been further optimized, leading to better performance in certain situations.
- HTML5 Support: PHP 8.4 has enhanced HTML5 support, making it easier to work with HTML documents.
- No Parentheses for new Operator: You can now create objects without parentheses when using the new operator, making the syntax simpler.
- DateTime from Unix Timestamp: PHP now allows you to directly create a
DateTime
object from a Unix timestamp, which simplifies date and time handling. - Multibyte String Functions: New functions like
mb_trim
,mb_ltrim
, andmb_rtrim
are added to handle multibyte strings more easily.