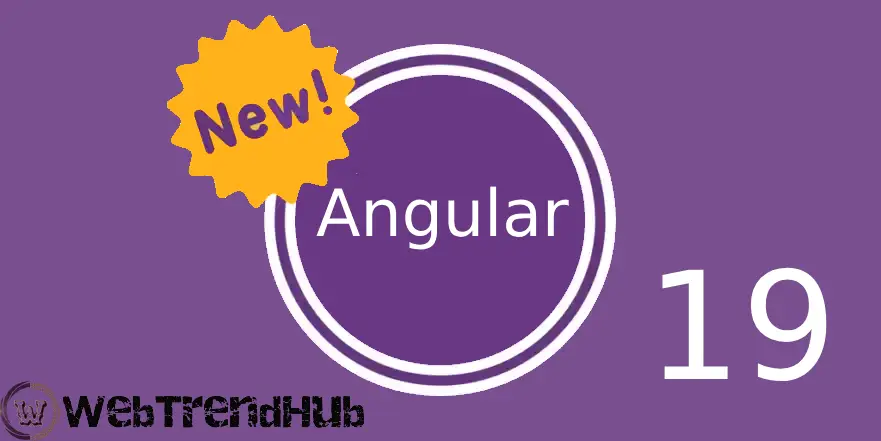
Angular 19, a major release focused on enhancing performance and improving the developer experience. With new features designed to make development faster and more efficient.The new update aims to streamline workflow and optimize application performance.
1. Incremental Hydration
What It Does: Only essential parts of a page are rendered initially, with additional parts loaded as needed, improving the speed.
// server.ts (server-side rendering with hydration)
const app = express();
app.get('/', (req, res) => {
res.send(renderToString(<App incrementalHydration={"true"} />));
});
When users visit a page, visible parts render instantly, and other sections load as they interact (e.g., scrolling).
2. Server Route Configuration
What It Does: Define how each route is rendered—server-side, client-side, or pre-rendered—for better control.
const routes: Routes = [
{ path: 'blog/:id', component: BlogComponent, renderMode: 'pre-render' },
{ path: 'product/:id', component: ProductComponent, renderMode: 'client' },
];
Here, blog routes can be pre-rendered for SEO, while dynamic pages like products load on the client.
3. Hot Module Replacement (HMR) for Styles and Templates
What It Does: Instantly see changes to styles or templates without reloading the page.
# Run the development server with HMR
ng serve --hmr
Modify a CSS file (e.g., change a button color) and watch the update live:
button {
background-color: blue; /* Change to green and see it instantly */
}
4. Zoneless Angular (Experimental)
What It Does: Removes the need for Zone.js, speeding up rendering and user interactions.
bootstrapApplication(AppComponent, {
providers: [
provideZoneLess(),
],
});
This enhances performance for applications with complex interactions.
5. Resource API
What It Does: Fetch and manage asynchronous data more efficiently.
import { Resource } from '@angular/core';
const productResource = new Resource(() => fetch('/api/products').then(res => res.json()));
@Component({
selector: 'app-products',
template: `<div *ngFor="let product of products">{{ product.name }}</div>`
})
export class ProductsComponent {
products = productResource.use();
}
This simplifies fetching and using data in components.
6. Enhanced CLI
What It Does: Speeds up builds and improves error messages.
# Run the development server
ng serve
You'll notice faster builds and clearer error outputs like:
ERROR: Cannot find module 'AppComponent'. Did you mean './AppComponent'?
With these features, Angular 19 makes development faster, easier, and more efficient! Try them out to see the improvements in action. 🚀