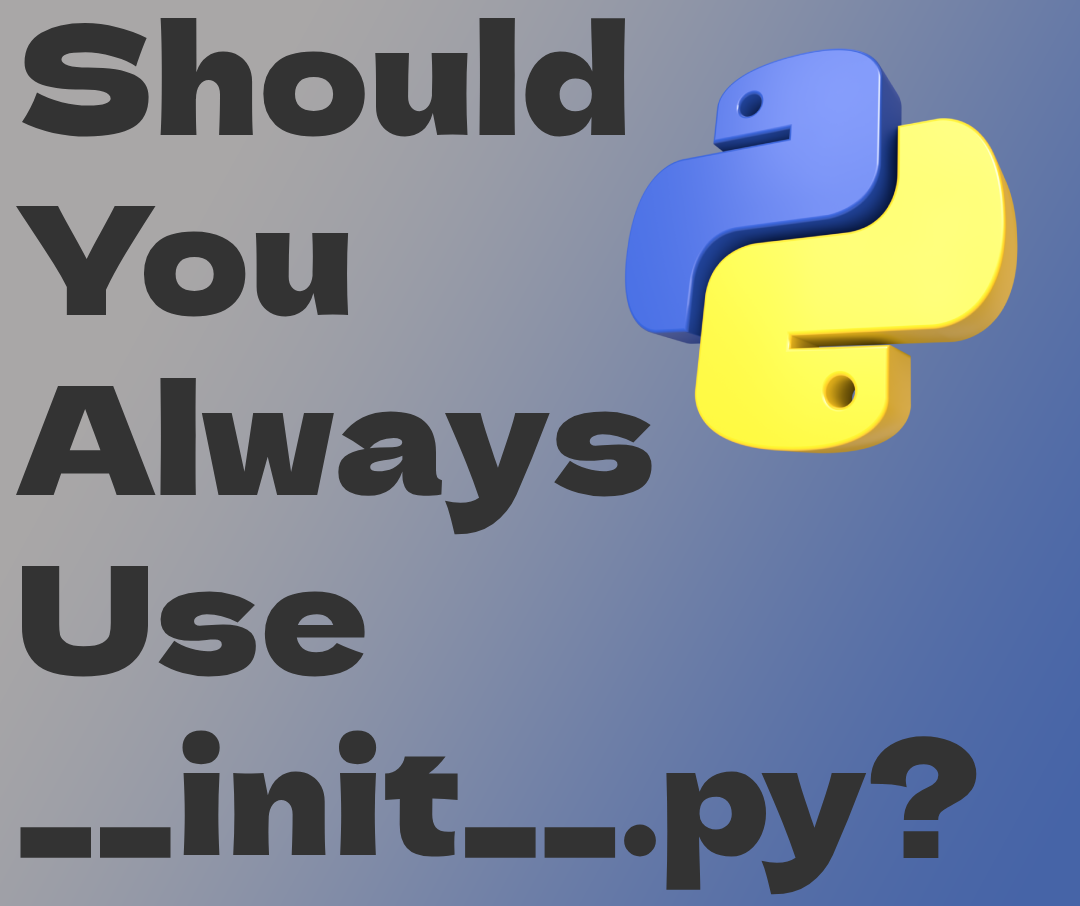
In today’s blog topic, we're going to be learning about __init__.py
and what it actually does in Python. If you've ever peeked into a Python project or a package directory, you may have noticed a file called __init__.py
. This little file is actually more important than it may look at first glance. Let’s break down its role and purpose in the simplest way possible.
So, What is __init__.py
?
__init__.py
is a special file in Python that signals to Python that a directory should be treated as a package. A package in Python is just a way of organizing related code into one place, making it easier to manage and use. Think of it like a folder on your computer that holds files related to a particular project or topic. When Python sees an __init__.py
file inside a directory, it treats that directory as a package, which means it can import and use code from there.
Why Do We Need __init__.py
?
In older versions of Python (before version 3.3), any folder you wanted to treat as a package had to contain an __init__.py
file. Without it, Python would ignore the folder, and you wouldn’t be able to import anything from it. Although it’s technically optional in more recent versions of Python, it’s still very common to include __init__.py
in a package. It helps keep your code organized and can provide additional functionality if needed.
What Can You Do Inside __init__.py
?
You might think that __init__.py
is just an empty file, but it can do a lot more if you need it to. Here are a few things you can use it for:
- Initialize Variables or Code: You can set up any initial values or even run code that should happen whenever the package is imported.
- Control Imports with
__all__
: Inside__init__.py
, you can define a special list called__all__
. This controls which modules (or files) should be available for import when someone imports the package. - Make Shortcuts for Imports: Instead of making users import every module separately, you can use
__init__.py
to create shortcuts, so importing the package brings in everything they might need right away.
Example of How __init__.py
Works
Let’s say you have a directory structure like this for a package called my_package
:
my_package/
│
├── __init__.py
├── module1.py
└── module2.py
Here’s how it might look in action:
# Inside __init__.py
from .module1 import some_function
from .module2 import another_function
__all__ = ["some_function", "another_function"]
This setup lets you do something like:
import my_package
my_package.some_function() # Can call directly if it's in __init__.py
Without __init__.py, this would throw an error because Python wouldn’t recognize my_package as an importable package.
Should You Always Use __init__.py? __init__.py is generally a good idea If you’re organizing a project with multiple modules that are related. It doesn’t need to be complex—many times, it’s just an empty file. But it keeps things organized and tells Python, “Hey, this folder is a package!”So, there you have it: a quick rundown of __init__.py and why it matters in Python projects. It may be a small file, but it plays a big role in helping your code stay organized and easy to manage.